Project Setup
I started by creating a root directory named tartarus-insight-rebuild
. Inside it, I created two subdirectories—one for each framework. The directory for Next.js is named nextjs
, and the directory for React Router is named react-router
.
tartarus-insight-rebuild
│── nextjs/ # Next.js project
│── react-router/ # React Router project
BashInside the nextjs
directory, I ran:
# From the nextjs directory
npx create-next-app@latest .
BashThe .
(dot) ensures that the project is created in the current directory.
During installation, I chose all the default options:
Would you like to use TypeScript? - Yes
Would you like to use ESLint? - Yes
Would you like to use Tailwind CSS? - Yes
Would you like your code inside a `src/` directory? - No
Would you like to use App Router? (recommended) - Yes
Would you like to use Turbopack for `next dev`? - Yes
Would you like to customize the import alias (`@/*` by default)? - No
BashSimilarly, for the React Router project, I ran:
# From the react-router directory
npx create-react-router@latest .
BashI accepted all the default options except for “Initialize a new git repository?”, where I chose No since I want both frameworks in a single repository. Instead, I will initialize Git at the root directory (tartarus-insight-rebuild
).
Before doing so, I need to remove the .git
folder from the nextjs
directory, as it was automatically initialized when running npx create-next-app
. Having nested Git repositories (a Git repository inside another Git repository) creates what’s called a Git submodule, which can be confusing and difficult to manage.
I’ll also delete the .gitignore
files from both the nextjs
and react-router
directories since I will maintain a single .gitignore
file in the root directory. This new .gitignore
file will combine the contents of the two deleted ones, with some adaptations to match our directory structure.
Steps to Clean Up and Initialize Git
1. Remove the .git
folder from the nextjs
directory:
# From the tartarus-insight-rebuild directory
rm -rf nextjs/.git
Bash2. Delete the .gitignore
files from both directories:
# From the tartarus-insight-rebuild directory
rm nextjs/.gitignore
rm react-router/.gitignore
Bash3. Initialize a Git repository in the root directory:
# From the tartarus-insight-rebuild directory
git init
Bash4. Add the new .gitignore
file at the root (a combination of the previous ones).
# See https://help.github.com/articles/ignoring-files/ for more about ignoring files.
# dependencies
**/node_modules
**/.pnp
**/.pnp.*
**/.yarn/*
!**/.yarn/patches
!**/.yarn/plugins
!**/.yarn/releases
!**/.yarn/versions
# testing
**/coverage
# next.js
**/nextjs/.next/
**/nextjs/out/
# React Router (Remix)
**/react-router/build/
**/.cache/
**/react-router/public/build/
# production
**/build/
**/dist/
# misc
.DS_Store
**/*.pem
**/.vscode/
**/.cache/
# debug
**/npm-debug.log*
**/yarn-debug.log*
**/yarn-error.log*
**/.pnpm-debug.log*
# env files (can opt-in for committing if needed)
**/.env*
!**/.env.example
# vercel
**/.vercel
# typescript
**/*.tsbuildinfo
**/next-env.d.ts
# Logs
**/logs
**/*.log
# Lock files (uncomment if you want to ignore these)
**/yarn.lock
**/pnpm-lock.yaml
**/package-lock.json
PlaintextA Quick Note on Git Initialization
Normally, you wouldn’t need to go through this cleanup process. If you were setting up these projects separately, you could just let each framework handle Git on its own:
- When creating the Next.js project, it automatically initializes a Git repository.
- When setting up React Router, you could choose Yes when asked to initialize a Git repository.
- Each project would also have its own
.gitignore
file.
However, because we want both frameworks in a single repository, we need to remove the extra .git
folders and .gitignore
files to avoid conflicts and manage everything from the root directory.
Running the Frameworks
The next step is to ensure that both frameworks run correctly.
1. Navigate to the nextjs
directory and start the development server:
# From the tartarus-insight-rebuild directory
cd nextjs
npm run dev
Bash2. Open your browser and go to http://localhost:3000/. You should see the default Next.js application.
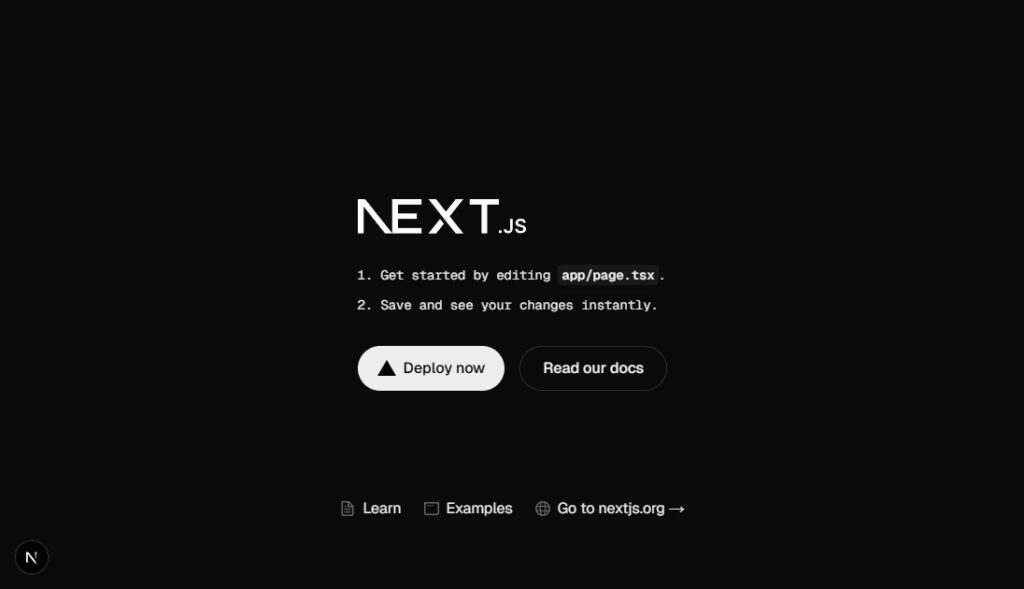
3. Open a new terminal, navigate to the react-router
directory, and start its development server:
# From the tartarus-insight-rebuild directory
cd react-router
npm run dev
Bash4. Visit http://localhost:5173/ in your browser to view the React Router application.
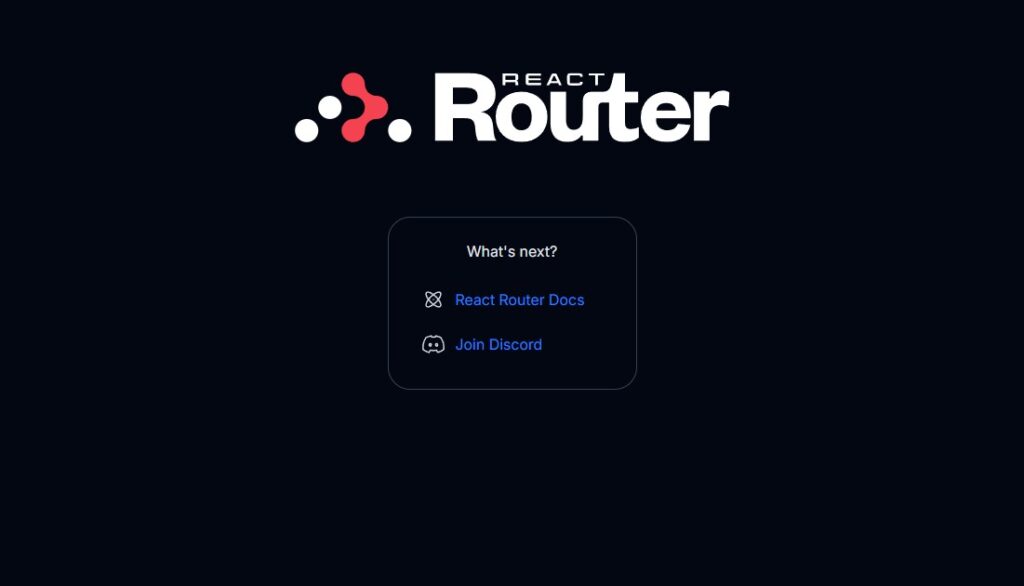
Cleaning Up Unnecessary Files
Now, let’s remove the extra files and code that we won’t be using in our projects.
Cleaning Up Next.js
Remove Default Public Assets
- Delete all files inside the
public
folder. - Replace
favicon.ico
in theapp
folder with our custom favicon.
Update Global Styles
- Open
globals.css
and delete everything except the first line:
/* nextjs > app > globals.css */
@import "tailwindcss";
CSSModify layout.tsx
- Keep the Google Fonts import (we will change this later).
- Update the
metadata
object:
// nextjs > app > layout.tsx
import type { Metadata } from "next";
import { Geist, Geist_Mono } from "next/font/google";
import "./globals.css";
const geistSans = Geist({
variable: "--font-geist-sans",
subsets: ["latin"],
});
const geistMono = Geist_Mono({
variable: "--font-geist-mono",
subsets: ["latin"],
});
export const metadata: Metadata = {
title: "Tartarus Insight",
description: "Trapped in the abyss of business struggles? Harness the Oracle's wisdom to ascend from struggle to success.",
};
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en">
<body
className={`${geistSans.variable} ${geistMono.variable} antialiased`}
>
{children}
</body>
</html>
);
}
TSXSimplify page.tsx
// nextjs > app > page.tsx
export default function Home() {
return (<h1>Tartarus Insight</h1>);
}
TSXCleaning Up React Router
Replace Default Favicon
- Inside the
public
folder, replacefavicon.ico
with our custom favicon.
Update Global Styles
- Open
app.css
and remove everything except:@import "tailwindcss";
/* react-router > app > app.css */
@import "tailwindcss";
CSSModify home.tsx
(Inside app/routes/
)
- Update the title and description with the same values as in Next.js:
// react-router > app > routes > home.tsx
import type { Route } from "./+types/home";
import { Welcome } from "../welcome/welcome";
export function meta({}: Route.MetaArgs) {
return [
{ title: "Tartarus Insight" },
{
name: "description",
content: "Trapped in the abyss of business struggles? Harness the Oracle's wisdom to ascend from struggle to success."
},
];
}
export default function Home() {
return <Welcome />;
}
TSXClean Up the Welcome Component
- Inside
app/welcome/
:- Delete
logo-dark.svg
andlogo-light.svg
. - Open
welcome.tsx
:- Remove the two import statements related to the deleted SVGs.
- Delete the
const resources
variable. - Replace the
return
statement with: <h1>Tartarus Insight</h1>
- Delete
// react-router > app > welcome > welcome.tsx
export function Welcome() {
return (<h1>Tartarus Insight</h1>);
}
TSXAnd that’s it! Both frameworks are now cleaned up.
Creating a GitHub Repository and Pushing the Code
Now that our project is set up and cleaned up, it’s time to create a GitHub repository and push our code.
1. Create a New GitHub Repository
- Go to GitHub and log in.
- Click on the New Repository button.
- I named the repository tartarus-insight-rebuild. You can name it whatever you want.
- Keep it public or private, depending on your preference.
- No need to initialize the repository with a README, .gitignore, or license—we already have a
.gitignore
file, and we’ll create the README and license later. - Click Create Repository.
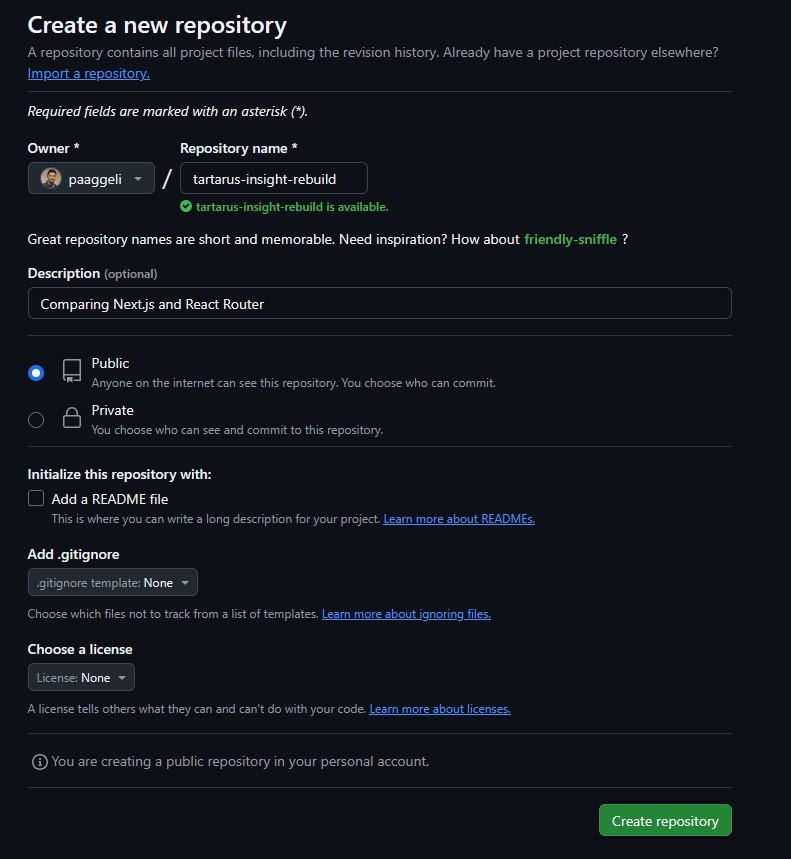
2. Check Your Current Git Branch
First, check which branch you are on:
git branch
BashIf it shows master
instead of main
, you can rename it:
git branch -m master main
Bash3. Stage and Commit Your Changes
Since we haven’t committed anything yet, let’s do that now:
git add .
git commit -m "Initial commit: Setup Next.js and React Router"
Bash4. Connect the Local Repository to GitHub
Now, link your local project to the GitHub repository you just created:
git remote add origin https://github.com/YOUR-USERNAME/your-repository-name.git
Bash5. Push Your Code to GitHub
If you renamed your branch to main
, push it like this:
git push -u origin main
BashIf you didn’t rename it and want to keep master
, you would instead run:
git push -u origin master
BashNow, refresh your GitHub repository page, and you should see all your files successfully uploaded! 🎉
What’s Next?
With our project now on GitHub, the next step is to start recreating the Tartarus Insight structure, focusing first on the home page layout and core components add Google Fonts to the apps. As I explore both frameworks, I’ll gradually build out the structure while learning how they handle components, styling, and functionality. 🚀
You can check out the Git repo here.
Leave a Reply